Fetching Player Flags #
Feature Flags are used in Experiments, Live Events and Feature Flag Variants to alter the game bahaviors to test or improve the player’s experience. Satori has wide range of options to allow you to fetch both user spesific values and default values of a feature flag via client APIs explained in client libraries.
Priority Order for Flag Values #
Different configurations in Satori can change the feature flag values assigned to the players. Satori uses following priority order to determine which value of the Feature Flag will be assigned to the player:
- Experiments
- Live Events
- Feature Flag Variants
If there are more than one experiments or live events, the one that is defined last will have the priority to determine the feature flag value. To warn you about these conditions, you will see following popup when you add more than one live event or experiment using the same feature flag:
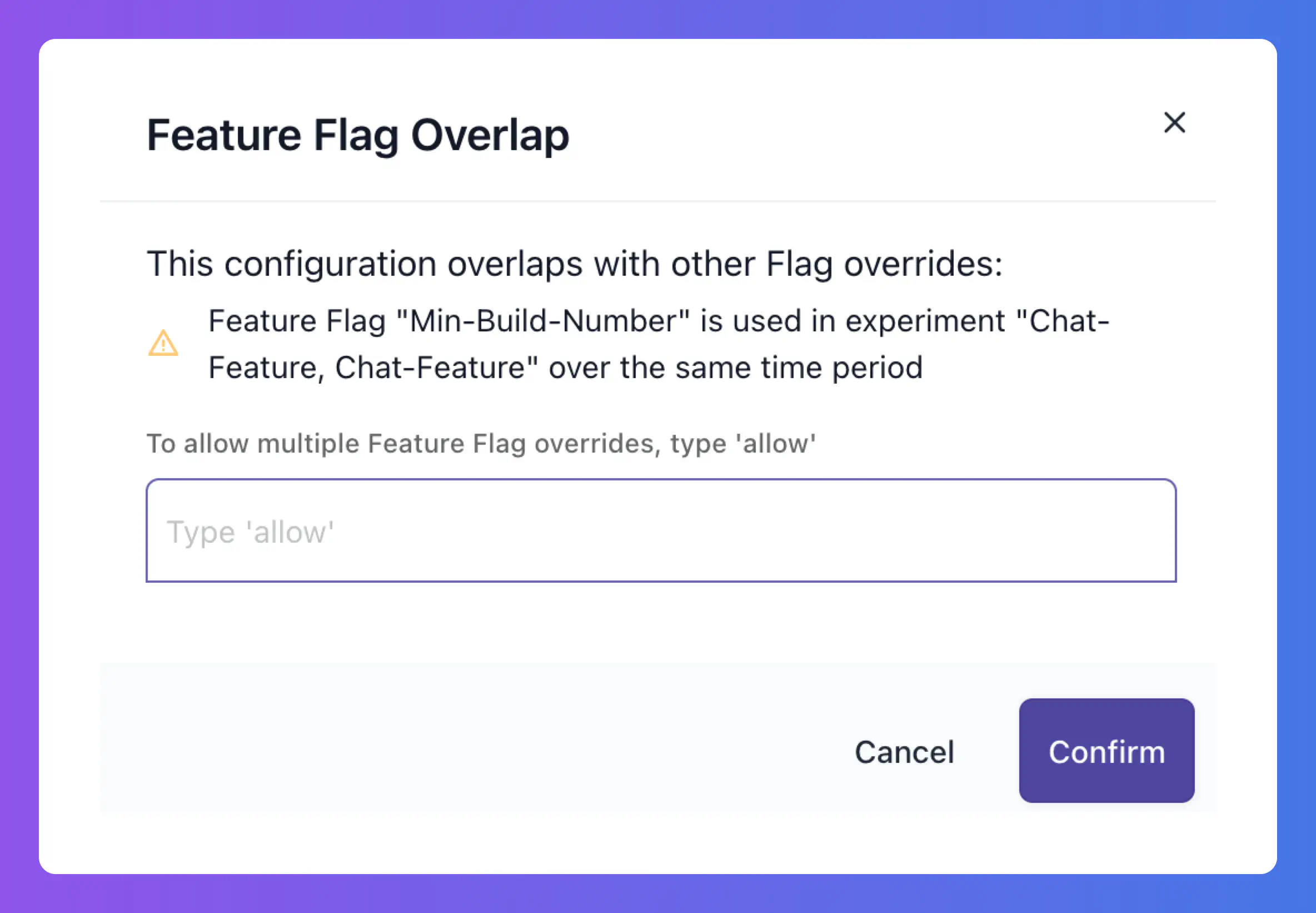
Fetching Player Flags #
To list the player’s flag values, you have two options:
- Fetch Player Flags using
GetFlagsAsync
: this endpoint will give your game client the value of the feature flags calculated based on the priority - Fetch Player Flags with Overrides using
GetFlagOverridesAsync
Fetch Flag Values for Identity #
GetFlagsAsync
endpoint will provide your game client the value of the feature flags assigned to the player by Satori based on the priority order explained in the previous section.
|
|
The response will include the feature flag value that is assigned to the player.
|
|
You will also receive additional information under change_reason
if the Feature Flag is changed from the default value.
|
|
Inside the change_reason
part:
type
will give you information about what changed the Feature Flag value"type": 1
means the value is changed by a Feature Flag Variant"type": 2
means the value is changed by a Live Event"type": 3
means the value is changed by an Experiment
name
will provide the name of the Satori feature that changed the flag value. For instance, in the example above, the name of the experiment is “Loot-Box-Effect-on-LTV”variant_name
will provide the variant name if available. In this example, the variant of the experiment that set the Flag value to 4 is “Variant-A—More-Loot-Boxes”
Fetch Flag Overrides for Identity #
If you want to get all of the available overrides and decide the priority your self - for instance your game may require a different stickiness approach, you can use the GetFlagOverridesAsync
endpoint.
|
|
Using this endpoint, you will receive all possible values for the player’s feature flags. Receiving all values, you can select which one to use for the player. Please note that using alternative values from this endpoint will not change which live event or experiment the player has joined. Metrics, live event and experiment results you see in the Satori Console won’t be aware of the value you choose to show to your player.
|
|
Overrides will be sent in an array and to understand each item in the array you can refer to following explanations:
type
parameter will give you information about the type of the override:"type": 1
Feature Flag Variant"type": 2
Live Events"type": 3
Live Event Flag Variant"type": 4
Experiments- If type is not available, it is the original value of flag
name
will provide the name of the Satori feature that overrides the flag value.variant_name
will provide the variant name, if available.value
is the flag value for this override.create_time_sec
is the UNIX timestamp that shows the creation time of the related Satori feature. This field can help you to decide if you want to manually set time based rules such using the newest flag value.